← All ExamplesHidden Browser Tab
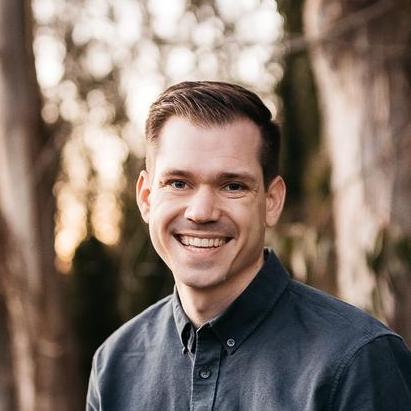
By Ryan Wiemer
Note: This example uses React with ES6 syntax.
class Hidden extends React.Component {
constructor(props) {
super(props)
this.state = {
title: 'Hidden Browser Tab - Subtle UI',
hiddenTitle: 'Come Back...'
}
}
componentDidMount() {
document.addEventListener('visibilitychange', this.handleVisibilityChange)
}
componentWillUnmount() {
document.removeEventListener('visibilitychange', this.handleVisibilityChange)
}
handleVisibilityChange = () => {
if (document.hidden) {
document.title = this.state.hiddenTitle
}
else {
document.title = this.state.title
}
}
render() {
return (<div/>)
}
}
const Instructions = () => {
return (
<div style={{
'width':'100%',
'height':'100%',
'padding':'1em',
'lineHeight': '1.4',
'background':'moccasin',
'textAlign':'center'
}}>
Click on a different browser tab to
see the title tag change
</div>
)
}
render (
<div>
<Hidden/>
<Instructions/>
</div>
)
Click on a different browser tab to see the title tag change
Demo
Considerations
- This ui pattern is primarily focused on desktop browsers where other tabs can easily be seen.