← All ExamplesScroll Loop
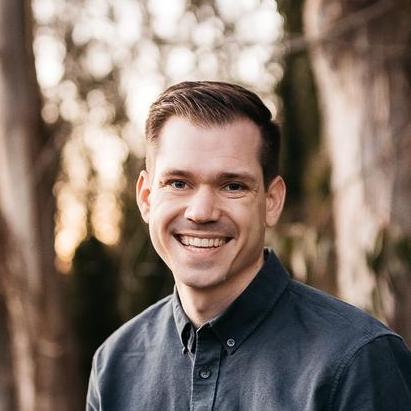
By Ryan Wiemer
Note: This example uses React with ES6 syntax and Styled Components.
const Loop = props => {
handleScroll = (e) => {
const bottom = e.target.scrollHeight
- e.target.scrollTop === e.target.clientHeight
if (bottom) {
e.target.scrollTop = 0
}
}
const Container = styled.section`
height: 500px;
overflow-y: scroll;
-webkit-overflow-scrolling: touch;
padding: 1em 1em 0 1em;
background: #eeeeee;
div {
display: flex;
align-items: center;
justify-content: center;
text-align: center;
font-size: 1.25em;
line-height: 1.4;
margin: 0 0 1em 0;
height: 500px;
background: lightblue;
}
`
return (
<Container onScroll={handleScroll}>
{props.children}
</Container>
)
}
render (
<Loop>
<div>↓ Scroll down the loop ↓</div>
<div>1</div>
<div>2</div>
<div>3</div>
<div>4</div>
<div>5</div>
</Loop>
)
↓ Scroll down the loop ↓
1
2
3
4
5
Demo
Considerations
- This particular ui pattern works best on desktop.
- Touch screen device scrolling can be tricky and inconsistent so it is common to see this ui pattern disabled on touch devices.
Inspiration